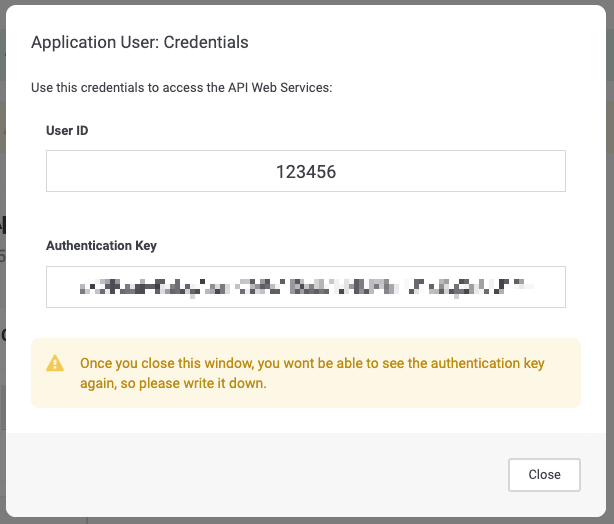
The REST API allows to create integrations, retrieve data and automate workflows. It accepts and returns JSON-encoded data, uses standard HTTP response codes and authentication using JSON Web Tokens.
The OpenAPI specification provides the standardized documentation of the API that both humans and computers are able to discover and understand. Check the Swagger documentation for more information.
To authenticate a request, a JSON Web Token is included as an Authorization
header. The token needs to be signed using an application user’s authentication key.
To be able to authenticate you need to create an application user first. You can do this in your account at Account > Users > Application Users.
After creating an application user, the user’s ID and authentication key will be shown to you. Please be aware, that once you close the dialog or browser window, you will not be able to see the authentication key again. So make sure to write it down.
Please refer to https://jwt.io/ to learn how to generate and sign JSON Web Tokens and to find corresponding libraries.
{
"alg": "HS256", // Defines the signing algorithm that is used, must be `HS256`
"type": "JWT", // The type of the token, must be JWT
"ver": 1 // The version of the JWT authorisation method
}
{
"sub": "123456", // The ID of the application user whose authentication key is used to sign the token
"iat": 1664375069, // The current UNIX timestamp in seconds, used to prevent https://en.wikipedia.org/wiki/Relay_attack
"requestPath": "/api/customers/search?order=lastname", // The path component of the requested URL, including any query string
"requestMethod": "GET" // The HTTP method of the request, as uppercase string
}
The JSON Web Token needs to be signed using your application user’s authentication key. Depending on the tool you are using to generate the token, you need to decode the authentication key, as you get it base64-encoded.
The generated JSON Web Token is included as a header in the request to the API.
GET /api/customers/search?order=lastname HTTP/1.1
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCIsInZlciI6MX0.eyJzdWIiOiIxMjM0NTYiLCJpYXQiOjE2NjQzNzUwNjkwNTksInJlcXVlc3RQYXRoIjoiL2FwaS9jdXN0b21lcnMvc2VhcmNoP29yZGVyPWxhc3RuYW1lIiwicmVxdWVzdE1ldGhvZCI6IkdFVCJ9.1QNdpsjZSnavrJkNM7aiAWHEXZS_o9Tnpg6pt3VDBUA
API methods return HTTP status codes to indicate success or failure. Some errors include an error code that allows to identify and handle the error programmatically. The response body to a failed request contains detailed information, see Error Response.
Status | Description |
---|---|
400 Bad Request |
The request was not accepted often due to missing or invalid parameters. |
401 Unauthorized |
The necessary authentication credentials are missing or incorrect. |
403 Forbidden |
The application user is missing the required permissions. |
404 Not Found |
The requested resource was not found. |
406 Not Acceptable |
The requested response format is not supported. |
409 Conflict |
The request conflicts with another request often because of to optimistic locking. |
413 Payload Too Large |
Too many operations in a bulk request. |
415 Unsupported Media Type |
The request provides unsupported or invalid data. |
416 Range Not Satisfiable |
The pagination offset exceeds the limit. |
422 Unprocessable Entity |
The request is well-formed but contains semantic errors. Check the response body for details. |
429 Too Many Requests |
Too many requests hit the API too quickly. |
5xx Errors |
An internal error occurred on the server. |
If an object is modified by multiple users or processes at the same time, this can lead to race conditions. To prevent this, the API uses optimistic locking.
An object that is potentially affected will have a version
property which gets incremented everytime the object is updated. When updating an object, its current version needs to be fetched and included in the update request.
If the version is missing or it does not match the current value, the update request will be declined and a response with HTTP status 409 Conflict
will be returned. In this case, the current version needs to be fetched and the update request sent again.
Many objects allow you to request additional information by expanding the response using the expand
request parameter. This parameter is available on many API methods like list, search, create and update.
Properties that can be expanded are marked accordingly in this documentation. There are two different kinds of expandable properties.
Linked objects are often represented by their IDs in API responses. These objects can be expanded using the expand
parameter to be fully included in the response.
Some available properties are not included in responses by default. They can be requested by using the expand
parameter.
Properties can be expanded recursively by specifying nested fields using a dot (.
). Expanding charge.transaction
will include the full charge
object as well as the full transaction
object. The maximum depth is at four levels.
Multiple properties can be expanded at once by specifying all of them in the expand
parameter separated by commas (,
).
expand=charge.transaction,labels
The same search query syntax is used accross the API for all search
API methods. The search query is passed in the query
parameter.
A search query is made up of terms, connectives, modifiers and comparators. The search grammar is expressed as EBNF with the following terminal symbols:
Item | Description |
---|---|
|
Any sequence of space, tab, or newline characters |
|
Any sequence of non-whitespace, non-special characters |
|
Any name, or any quoted string (single or double quotes are both permitted) |
Query = Term { [ whitespace Connective ] whitespace Term }
A query consists of one or more terms separated by whitespace or single connectives.
Connective = "AND" | "OR"
If no connective is specified between two terms, AND
is implied.
Term = [ Modifier ] ( "(" Query ")" | name Comparator [ value ] )
A term consists of an optional modifier, followed by either a subquery enclosed in parantheses, or a name, comparator and value.
Values can be quoted (single or double quotes). If the value does not contain any whitespace, the quotes are optional.
Modifier = "-" | "NOT" whitespace
-
with no following whitespace and NOT
followed by whitespace mean the same thing.
Comparator = ":" | ":<" | ":>" | ":<=" | ":>=" | ":*" | ":~"
Comparator | Description |
---|---|
|
Equals (case-insensitive) |
|
Less than |
|
Greater than |
|
Less than or equal |
|
Greater than or equal |
|
Is |
|
Contains |
query=firstName:"John Peter" lastName:Doe
Search for John Peter
as first name AND Doe
as last name
query=charge.transaction.state=PENDING AND currency=EUR
Search for objects linked to pending transactions AND with currency EUR
query=quantity:>2 quantity:<=10
Search for objects with quantities between 3 and 10.
query=NOT currency:EUR
query=-currency:EUR
Search that excludes objects with currency EUR
.
query=state:SUCCESSFUL AND (currency:CHF OR currency:EUR)
Search for successful objects with currency CHF
or EUR
query=paymentMethod:*
Search for objects without a payment method.
The Is NULL
comparator (:*
) does not ask for a value.
query=color:~red,blue,green
Search for objects with all the colors red
, blue
and green
.
The objects retrieved from a search
API method can be sorted by specifying the order
parameter. They can be ordered by multiple fields.
order=lastName
order=lastName+firstName
order=lastName:ASC+firstName:DESC
The fields are separated by a space. The order (ASC
or DESC
) can be explicitly specified by adding it after a :
. If no order is specified, ASC
is implied.
The response of a list
API method represents a single page of objects. The parameters limit
, before
, after
and order
can be used to control pagination. If you specify none of them, you will receive the first 10 objects in chronological order.
You can set the after
parameter to an object’s ID to retrieve the page of objects coming immediately after the named object in the applied order. Similarly, you can use the before
parameter to retrieve the objects coming immediately before the named object. Both parameters after
and before
can be set at the same time to get a specific range of objects.
Use the limit
parameter to specify the maximum number of objects that should be returned. This cannot exceed 100.
The response of a search
API method represents a single page of filtered objects. The parameters limit
and offset
can be used to control pagination. If you specify none of them, you will receive the first 10 objects.
The offset
parameter allows you to get objects at an offset. So if you set offset
to 20, the first 20 objects will be skipped and the first object returned will be the 21st.
Use the limit
parameter to specify the maximum number of objects that should be returned. This cannot exceed 100.
Some objects have a metadata
property. You can use this property to attach arbitrary key-value data to these objects. Metadata can be used for storing additional, structured information on an object. Metadata is not used or changed by the system.
You can specify up to 25 keys, with key names up to 40 and values up to 512 characters long.
Do not store any sensitive information (bank account numbers, card details, passwords, …) in metadata.
Our REST API follows a versioned approach to support evolving functionality while maintaining backward compatibility. Each version of the API is identified in the URL path (e.g., /v2.0/
, /v2.1/
, /v3.0/
, etc.).
New API versions may introduce breaking changes, such as modified request/response formats, changed behavior, or removed endpoints. These changes are made to improve performance, security, or to simplify the overall API experience.
Older versions of the API will continue to function as-is without requiring any changes on your end. However, we recommend migrating to the latest version to take advantage of new features and enhancements.
When an endpoint is removed in a newer version, requests to it will return a 410 Gone
response. If a replacement is available, it will be documented accordingly.
You can view a complete list of deprecated operations and their replacements here: Deprecated REST API Operations
This section details all API services and their operations. An operation may accept data as part of the path, in the request’s header, as query parameter or in the request’s body.
Here is an example request to update a customer:
POST /api/v2/customers/123?expand=metaData HTTP/1.1
Space: 1
Authorization: Bearer [calculated JWT token]
{"version": 1, "givenName": "Jane", "familyName": "Doe"}
Permanently deletes a payment connector configuration. It cannot be undone.
Initiates a payment terminal transaction and waits for its completion. If a timeout occurs, retrying will resume the transaction from where it left off.
90 seconds
Initiates a payment terminal transaction and waits for its completion. If a timeout occurs, retrying will resume the transaction from where it left off.
90 seconds
Generates temporary transaction credentials to delegate access to the REST API for the specified transaction.
60 seconds
Processes the transaction using the provided card details, potentially utilizing 3-D Secure. Returns a URL where the buyer must be redirected to complete the transaction.
Assigns the provided token to the transaction, processes it, and returns a URL for customer redirection to complete the transaction.
Export human users into a CSV file.
60 seconds
Confirm the installation of a web app. This has to be done when the user returns to the web app after granting permissions, using the activation code provided in the request.
This section details all models that are accepted or returned by API operations.
Represents the result of a single operation in a bulk request.
A document template contains the customizations for a particular document template type.
A dunning condition determines which dunning flow is applied on a dunning case.
A manual task requires the manual intervention of a human.
The manual task type indicates what kind of manual task is required to be executed by the human.
Payment processors serve as intermediaries that establish connections with third-party companies, known as payment service providers. These providers are responsible for managing the technical aspects of payment transactions, ensuring seamless and secure payment processing.
A connector condition defines criteria that a transaction must meet for a connector configuration to process the payment.
A payment information hash is generated from user input, ensuring consistent and collision-free results for identical inputs.
A refund is a credit issued to the customer, which can be initiated either by the merchant or by the customer as a reversal.
The confirmation response provides details about the installation of the web app.
The webhook identity represents a set of keys that will be used to sign the webhook messages.